NSAttributedString 文本属性详解
NSAttributedString包含的所有Key
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
| NSFontAttributeName; //字体,value是UIFont对象
NSParagraphStyleAttributeName; //绘图的风格(居中,换行模式,间距等诸多风格),value是NSParagraphStyle对象
NSForegroundColorAttributeName; // 文字颜色,value是UIFont对象
NSBackgroundColorAttributeName; // 背景色,value是UIFont
NSLigatureAttributeName; // 字符连体,value是NSNumber
NSKernAttributeName; // 字符间隔
NSStrikethroughStyleAttributeName; //删除线,value是NSNumber
NSUnderlineStyleAttributeName; //下划线,value是NSNumber
NSStrokeColorAttributeName; //描绘边颜色,value是UIColor
NSStrokeWidthAttributeName; //描边宽度,value是NSNumber
NSShadowAttributeName; //阴影,value是NSShadow对象
NSTextEffectAttributeName; //文字效果,value是NSString
NSAttachmentAttributeName; //附属,value是NSTextAttachment 对象
NSLinkAttributeName; //链接,value是NSURL or NSString
NSBaselineOffsetAttributeName; //基础偏移量,value是NSNumber对象
NSUnderlineColorAttributeName; //下划线颜色,value是UIColor对象
NSStrikethroughColorAttributeName; //删除线颜色,value是UIColor
NSObliquenessAttributeName; //字体倾斜
NSExpansionAttributeName; //字体扁平化
NSVerticalGlyphFormAttributeName; //垂直或者水平,value是 NSNumber,0表示水平,1垂直
|
第一步创建UILabel及NSMutableAttributedString的实例对象,之后的效果改变都是作用在它们上面:
1
2
3
4
5
6
7
| UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, self.view.bounds.size.width - 40, 50)];
label.textAlignment = NSTextAlignmentCenter;
label.center = self.view.center;
[self.view addSubview:label];
NSMutableAttributedString *attributedString = [[NSMutableAttributedString alloc] initWithString:@"caiiiac.github.io"];
|
字体 颜色 背景色
NSFontAttributeName
NSForegroundColorAttributeName
NSBackgroundColorAttributeName
效果
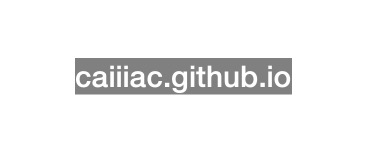
代码
1
2
3
4
5
6
| NSDictionary * attris = @{NSForegroundColorAttributeName:[UIColor whiteColor],
NSBackgroundColorAttributeName:[UIColor grayColor],
NSFontAttributeName:[UIFont boldSystemFontOfSize:30]};
[attributedString setAttributes:attris range:NSMakeRange(0,attributedString.length)];
label.attributedText = attributedString;
|
下划线
NSUnderlineStyleAttributeName
NSUnderlineColorAttributeName
效果
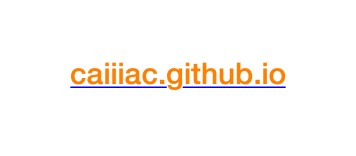
代码
1
2
3
4
5
6
7
| NSDictionary * attris = @{NSFontAttributeName:[UIFont boldSystemFontOfSize:30],
NSForegroundColorAttributeName:[UIColor orangeColor],
NSUnderlineStyleAttributeName:@(NSUnderlineStyleSingle),
NSUnderlineColorAttributeName:[UIColor blueColor],};
[attributedString setAttributes:attris range:NSMakeRange(0,attributedString.length)];
label.attributedText = attributedString;
|
描边
NSStrokeColorAttributeName
NSStrokeWidthAttributeName
效果
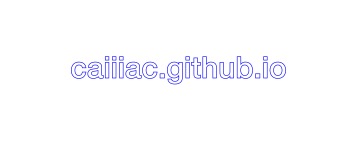
代码
1
2
3
4
5
6
7
| NSDictionary * attris = @{NSFontAttributeName:[UIFont boldSystemFontOfSize:30],
NSForegroundColorAttributeName:[UIColor whiteColor],
NSStrokeColorAttributeName:[UIColor blueColor],
NSStrokeWidthAttributeName:@(2)};
[attributedString setAttributes:attris range:NSMakeRange(0,attributedString.length)];
label.attributedText = attributedString;
|
阴影
效果
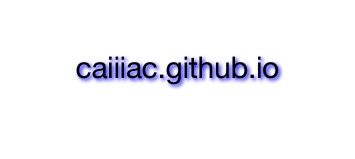
代码
1
2
3
4
5
6
7
8
9
| NSShadow * shadow = [[NSShadow alloc] init];
shadow.shadowColor = [UIColor blueColor];
shadow.shadowBlurRadius = 4.0;
shadow.shadowOffset = CGSizeMake(2.0, 2.0);
NSDictionary * attris = @{NSFontAttributeName:[UIFont systemFontOfSize:30],
NSShadowAttributeName:shadow};
[attributedString setAttributes:attris range:NSMakeRange(0,attributedString.length)];
label.attributedText = attributedString;
|
字符间隔
默认间隔
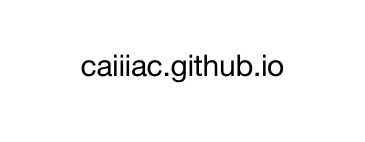
间隔为5
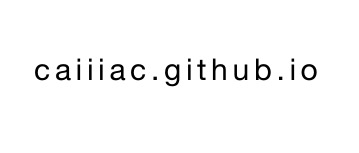
代码
1
2
3
4
5
| NSDictionary * attris = @{NSKernAttributeName:@(5),
NSFontAttributeName:[UIFont systemFontOfSize:30]};
[attributedString setAttributes:attris range:NSMakeRange(0,attributedString.length)];
label.attributedText = attributedString;
|
字体倾斜
NSObliquenessAttributeName
效果
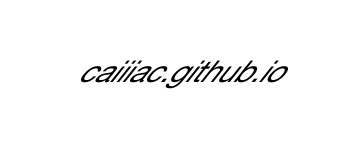
代码
1
2
3
4
5
| NSDictionary * attris = @{NSObliquenessAttributeName:@(0.8),
NSFontAttributeName:[UIFont systemFontOfSize:30]};
[attributedString setAttributes:attris range:NSMakeRange(0,attributedString.length)];
label.attributedText = attributedString;
|
字体扁平化
效果
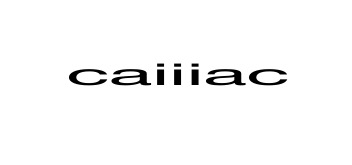
代码
1
2
3
4
5
| NSDictionary * attris = @{NSExpansionAttributeName:@(1.0),
NSFontAttributeName:[UIFont systemFontOfSize:30]};
[attributedString setAttributes:attris range:NSMakeRange(0,attributedString.length)];
label.attributedText = attributedString;
|
添加图片
NSAttachmentAttributeName
效果
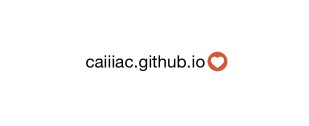
代码
1
2
3
4
5
6
7
8
| NSTextAttachment * attach = [[NSTextAttachment alloc] init];
attach.image = [UIImage imageNamed:@"收藏后"];
attach.bounds = CGRectMake(2, -4, 20, 20);
NSAttributedString * imageStr = [NSAttributedString attributedStringWithAttachment:attach];
[attributedString appendAttributedString:imageStr];
label.attributedText = attributedString;
|
绘图风格
效果
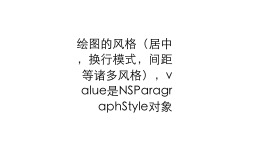
代码
自定义TextView类
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
| @implementation TextView
- (void)drawRect:(CGRect)rect {
// Drawing code
NSMutableAttributedString * attributeStr = [[NSMutableAttributedString alloc] initWithString:@"绘图风格(居中,换行模式,间距等诸多风格),value是NSParagraphStyle对象"];
NSMutableParagraphStyle * paragraphStyle = [[NSMutableParagraphStyle alloc] init];
paragraphStyle.alignment = NSTextAlignmentRight;
paragraphStyle.headIndent = 4.0;
paragraphStyle.lineBreakMode = NSLineBreakByCharWrapping;
paragraphStyle.lineSpacing = 2.0;
NSDictionary * attributes = @{NSParagraphStyleAttributeName:paragraphStyle};
[attributeStr setAttributes:attributes range:NSMakeRange(0, attributeStr.length)];
[attributeStr drawInRect:self.bounds];
}
@end
|
ViewController中添加代码
1
2
3
4
| TextView *textView = [[TextView alloc] initWithFrame:CGRectMake(0, 0, 100, 80)];
textView.backgroundColor = [UIColor whiteColor];
textView.center = CGPointMake(self.view.center.x, self.view.center.y + 30);
[self.view addSubview:textView];
|
Demo地址:点此查看